Converter
With a converter, you can apply Javascript to any of your previously created data sources. This is especially helpful if your data source returns the data in a structure that is not optimal for your use case.
[Note: This feature is still in BETA ]
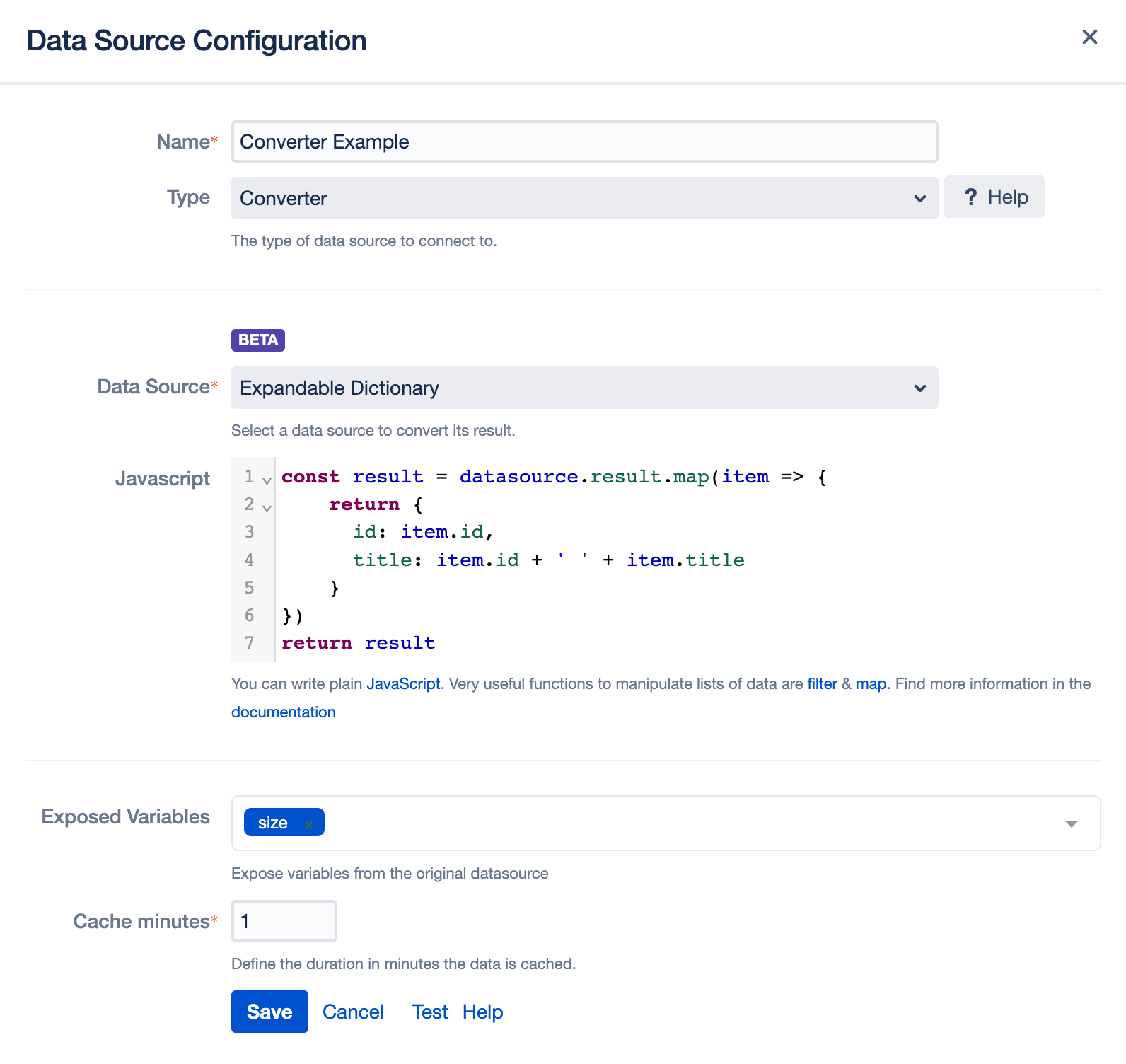
Create Data Source
Create a new data source of Converter
type and select up to 3 data sources whose data will be converted.
Javascript
Now you can add JavaScript that will convert the data as needed.
Let’s assume the underlying data source returns the following JSON
{
"result": [
{
"id": 1,
"title": "This is a product"
},
{
"id": 2,
"title": "Another product"
}
]
}
To access the underlying data source you can use the variable datasource
. This is also the simplest possible conversion script that just returns back the original:
return datasource
A more complex script that transforms the result could look like this:
return datasource.result.map(item => item.id + ' ' + item.title)
This will return the following JSON:
[
"1 This is a product",
"2 Another product"
]
To get a more usable result you could change the example as follows:
return datasource.result.map(item => ({
id: item.id,
title: item.id + ' ' + item.title
}))
This will return the following JSON:
[
{
"id": 1,
"title": "1 This is a product"
},
{
"id": 2,
"title": "2 Another product"
}
]
Find more details how to use JavaScript in general here.
Very useful functions to manipulate lists of data are filter & map like shown in the example above.
Access Multiple Data Sources
If you have chosen more than one data source, you can access each of them using the array accessor. Here's an example:
const result1 = datasource[0].result.map(item => item.id + ' ' + item.title)
const result2 = datasource[1].result.map(item => item.id + ' ' + item.title)
return result1.concat(result2)
In this example datasource[0]
and datasource[1]
are used to access each source.
Exposed Variables
This selection allows you to expose variables existing in your original data source.
Limitations
The original data source including the conversion script cannot exceed a maximum of 6 MB
The converted data cannot exceed a maximum of 6 MB
The script must return a result within 3 seconds
The conversion script itself cannot expose any variables. This might change in the future.
You cannot access contextual values like $.user or $.issue in the conversion script. This might change in the future