Template Syntax
Top-Level List Loop
Given the following JSON data, which is often the result when using a context filter, that returns a top level list:
[
{
"id": 1,
"name": "Mario Speedwagon",
"username": "mario"
},
{
"id": 2,
"name": "Anna Sthesia",
"username": "anna"
}
]
You can access the list in the template by using the following syntax. {{#.}}
to start the loop and {{/.}}
to close the loop.
<table class="aui aui-table-list">
<thead>
<th>ID</th>
<th>Name</th>
</thead>
<tbody>
{{#.}}
<tr>
<td>{{ id }}<td>
<td>{{ name }}<td>
</tr>
{{/.}}
</tbody>
</table>
Named List Loop
Given the following JSON data, which does not return a top level list, but has to be accessed by name:
{
"result": [
{
"id": 1,
"name": "Mario Speedwagon",
"username": "mario"
},
{
"id": 2,
"name": "Anna Sthesia",
"username": "anna"
}
]
}
You can access the list in the template by using the following syntax. {{#result}}
to start the loop and {{/result}}
to close the loop.
<table class="aui aui-table-list">
<thead>
<th>ID</th>
<th>Name</th>
</thead>
<tbody>
{{#result}}
<tr>
<td>{{ id }}<td>
<td>{{ name }}<td>
</tr>
{{/result}}
</tbody>
</table>
Number Formatting
You can use helper functions for easier formatting of numbers.
The following examples are run on this JSON:
{
"number1": 1.5,
"number2": 1000.457
}
Examples
{{ FormatCurrency number1 }}
-> $1.50
{{ FormatNumber number1 'N0' }}
-> 2
{{ FormatNumber number1 'N1' }}
-> 1.5
{{ FormatNumber number1 'N2' }}
-> 1.50
The default setting for the number formatting is en-US
. If you need other formatting options, you can easily add another Format Culture:
{{ FormatCurrencyCulture number1 'de-DE'}}
-> 1,50 €
{{ FormatNumberCulture number2 'N2''de-DE' }}
-> 1.000,46
Date & Time Formatting
Helper functions can help you with basic date and time formatting:
{
"time": "2020-09-01T22:05:45.488+02:00"
}
Examples
{{ FormatDate time 'g''en-Us'}}
-> "9/1/2020 10:05 PM"
{{ OffsetTimezoneThenFormatDate time '+8''g''en-AU'}}
-> "2/9/2020 4:05 am"
The g
in the examples is a format type that can be changed to the following formats. This variable varies depending on the Format Culture.
* d :08/17/2000
* D :Thursday, August 17, 2000
* f :Thursday, August 17, 2000 16:32
* F :Thursday, August 17, 2000 16:32:32
* g :08/17/2000 16:32
* G :08/17/2000 16:32:32
* m :August 17
* r :Thu, 17 Aug 2000 23:32:32 GMT
* s :2000-08-17T16:32:32
* t :16:32
* T :16:32:32
* u :2000-08-17 23:32:32Z
* U :Thursday, August 17, 2000 23:32:32
* y :August, 2000
Parsing Custom Date-Time Texts
If you have a date-time value in text form that is not automatically recognized, you need to explicitly define the expected format structure to help the parser interpret it correctly.
This is especially important when working with locales, uncommon date layouts, or strings without delimiters.
Suppose you have a time in the following format:
{
"time": "Fri Apr 11 16:30:21 UTC 2025"
}
You should define the format using a pattern, for example:
{{ FormatDateFromText time 'ddd MMM dd HH:mm:ss UTC yyyy' 'g' 'en-Us }}
-> "11/4/2025 16:30 PM"
IF / ELSE Example
If you want to display a coloured badge depending on whether a value is true or false, consider the following JSON data…
[
{
"isActive": false,
"company": "POWERNET"
},
{
"isActive": true,
"company": "EXIAND"
},
{
"isActive": false,
"company": "KYAGURU"
},
{
"isActive": true,
"company": "OATFARM"
},
{
"isActive": true,
"company": "VIAGRAND"
}
]
…in combination with the following template (with {{#isActive}}
being the IF
part and {{^isActive}}
referring to the ELSE
part).
<table class="aui">
{{#.}}
<tr>
<td>{{company}}</td>
<td>
{{#isActive}}
<span class="aui-lozenge aui-lozenge-removed">Inactive</span>
{{/isActive}}
{{^isActive}}
<span class="aui-lozenge aui-lozenge-success">Active</span>
{{/isActive}}
</td>
</tr>
{{/.}}
</table>
The result should look like this:
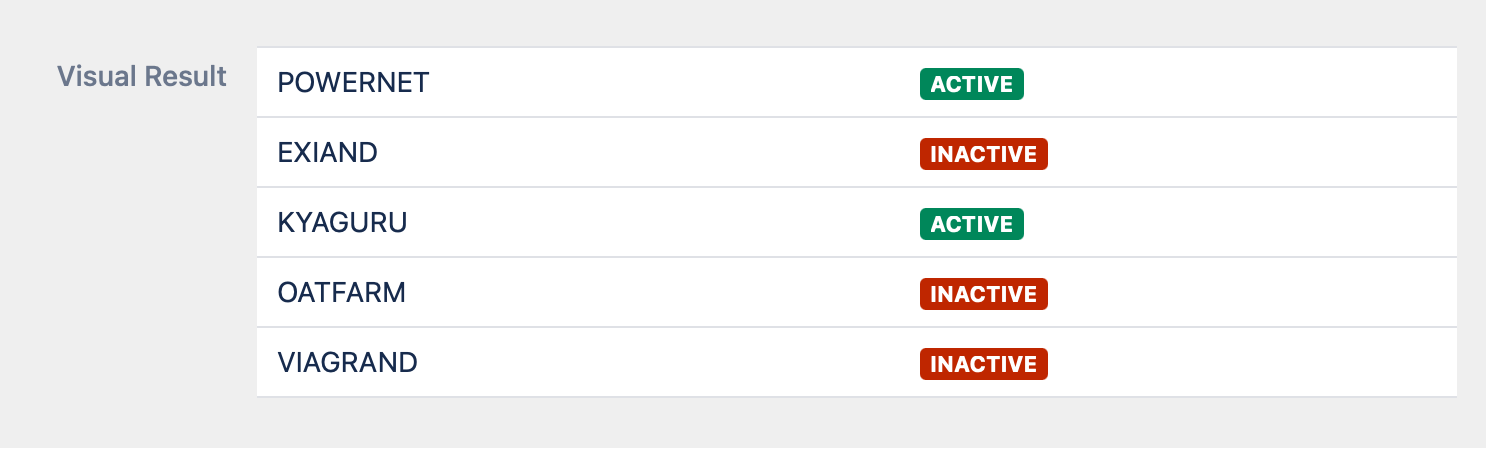
In case you need to compare the value of your data source, you can use the following helper function to achieve a similar result:
<table class="aui">
{{#.}}
<tr>
<td>{{company}}</td>
<td>
<span class="aui-lozenge aui-lozenge-{{ RenderOnMatch isActive 'true''success''removed'}}">
{{ RenderOnMatch isActive 'true''Active''Inactive'}}
</span>
</td>
</tr>
{{/.}}
</table>
RenderOnMatch Example Explained
{{ RenderOnMatch field 'compare''equal''notequal'}}
This helper function compares the value compare
with the value of the field
and renders equal
if they are identical and notequal
if they differ.
SearchReplace
In case you have a text that contains line breaks like \n
or \r\n
and want to display those line breaks in HTML you can use the following syntax:
{{ SearchReplace field '\n''<br>' }}
or
{{ SearchReplace field '\r\n''<br>' }}
UrlEncode
When constructing a URL that may include special characters, it's essential to encode these characters to ensure the URL functions correctly. To achieve this, use the UrlEncode
syntax:
<a href="https://my.url.com?query={{ UrlEncode field }}>My Link</a>
This encoding ensures that special characters in your field
are converted into a format that is safe and recognizable within a URL.
Reload - Button
If you want to provide users with the ability to manually refresh the information panel, you can add a reload button using a specific CSS class. This button will function as a refresh trigger. Here are two examples of how you can implement this:
Anchor Tag Example:
<a href="#" class="ed-refresh">Reload</a>
Button Tag Example:
<button class="aui-button ed-refresh">Reload</button>
By including the ed-refresh
class, the button or link will allow users to refresh the panel's content manually.
Arithmetics
There are also helper functions to do basic arithmetics in combination with formatting of the result.
The following examples are run on this JSON
{
"number1": 6,
"number2": 3
}
Examples
You can add, subtract, divide and multiple decimal values.
{{ AddThenFormat number1 number2 'N1' }}
-> 9.0
{{ SubThenFormat number1 number2 'N1' }}
-> 3.0
{{ DivThenFormat number1 number2 'P1' }}
-> 50.0%
{{ MulThenFormat number2 number1 'N1' }}
-> 18.0
If you need different culture formats you can customize them as well. The default setting for number formatting is en-US
.
{{ AddThenFormatCulture number1 number2 'N1''de-DE' }}
-> 9,0
{{ SubThenFormatCulture number1 number2 'N1''de-DE' }}
-> 3,0
{{ DivThenFormatCulture number1 number2 'P2''de-DE' }}
-> 50,00%
{{ MulThenFormatCulture number2 number1 'N1''de-DE' }}
-> 18,0
Access Array by Index
The following examples are run on this JSON:
{
"result":[
{ "name" : "anybody"},
{ "name" : "nobody"}
]
}
Examples
{{#result.0}} {{ name }} {{/result.0}}
-> anybody
{{#result.1}} {{ name }} {{/result.1}}
-> nobody
{{ AccessArrayByIndex 'result''0''name' }}
-> anybody
{{ AccessArrayByIndex 'result''1''name' }}
-> nobody
The following examples are run on this JSON:
[
{ "name" : "anybody"},
{ "name" : "nobody"}
]
Examples
{{ AccessArrayByIndex '.''0''name' }}
-> anybody
{{ AccessArrayByIndex '.''1''name' }}
-> nobody
Access Array by Condition
Sometimes you need to access specific values of an array by condition index of an index. This can be achieved with this function.
The following examples are run on this JSON:
{
"result":[
{ "id": 123, "name" : "anybody"},
{ "id": 345, "name" : "nobody"}
]
Examples
{{ AccessArrayByCondition 'result''$..[?(@.id==123)]''$.name' }}
-> anybody
{{ AccessArrayByCondition 'result''$..[?(@.id==345)]''$.name' }}
-> nobody
Javascript
Due to security reasons, we currently don’t allow any custom Javascript logic within templates. However, you can still work with AUI elements that don’t need extra scripting, like the expander.